#include<stdio.h>
#include<stdlib.h>
struct node
{
int c;
int exp;
struct node *next;
};
main()
{
struct node *start=NULL,*start1=NULL,*start2=NULL;
int n,m;
void create(struct node **, int);
void show(struct node *);
void add(struct node *,struct node*,struct node**);
printf("\nEnter the number of elements you want in your first polynomial :");
scanf("%d",&m);
create(&start,m);
printf("\nEnter the number of elements you want in your second polynomial :");
scanf("%d",&n);
create(&start1,n);
add(start,start1,&start2);
printf("\n first linked list is :\n");
show(start);
printf("\n second linked list is :\n");
show(start1);
printf("\n added linked list is :\n");
show(start2);
getch();
}
void create(struct node **q,int n)
{
int i;
struct node *lst;
struct node *p;
lst=NULL;
printf("\nEnter the data only in the correct polynomial form :\n ");
for(i=1;i<=n;i++)
{
p=(struct node *)malloc(sizeof(struct node));
printf("\nEnter the %d cofficient :",i);
scanf("%d",&(p->c));
printf("\nEnter the %d exponent :",i);
scanf("%d",&(p->exp));
p->next=NULL;
if(*q==NULL)
*q=p;
else
lst->next=p;
lst=p;
}
}
void show(struct node *start)
{
while(start!=NULL)
{
printf(" %dX%d",start->c,start->exp);
start=start->next;
}
}
void add(struct node *start,struct node *start1,struct node **start2)
{
struct node *ptr1,*ptr2,*ptr3=NULL,*p,*lst;
ptr1=start;
ptr2=start1;
while(ptr1!=NULL&&ptr2!=NULL)
{
p=(struct node*)malloc(sizeof(struct node));
p->next=NULL;
if(ptr1->exp==ptr2->exp)
{
p->exp=ptr1->exp;
p->c=ptr1->c+ptr2->c;
ptr1=ptr1->next;
ptr2=ptr2->next;
}
else if(ptr1->exp>ptr2->exp)
{
p->exp=ptr1->exp;
p->c=ptr1->c;
ptr1=ptr1->next;
}
else
{
p->exp=ptr2->exp;
p->c=ptr2->c;
ptr2=ptr2->next;
}
if(ptr3==NULL)
{
ptr3=p;
}
else
{
lst->next=p;
}
lst=p;
}
if(ptr1==NULL)
{
while(ptr2!=NULL)
{
p=(struct node*)malloc(sizeof(struct node));
p->next=NULL;
p->exp=ptr2->exp;
p->c=ptr2->c;
ptr2=ptr2->next;
if(ptr3==NULL)
{
ptr3=p;
}
else
{
lst->next=p;
}
lst=p;
}
}
else if(ptr2==NULL)
{
while(ptr1!=NULL)
{
p=(struct node*)malloc(sizeof(struct node));
p->next=NULL;
p->c=ptr1->c;
p->exp=ptr1->exp;
ptr1=ptr1->next;
if(ptr3==NULL)
{
ptr3=p;
}
else
{
lst->next=p;
}
lst=p;
}
}
*start2=ptr3;
}
Monday, July 11, 2011
Write a program to find polynomial addition using linked list.
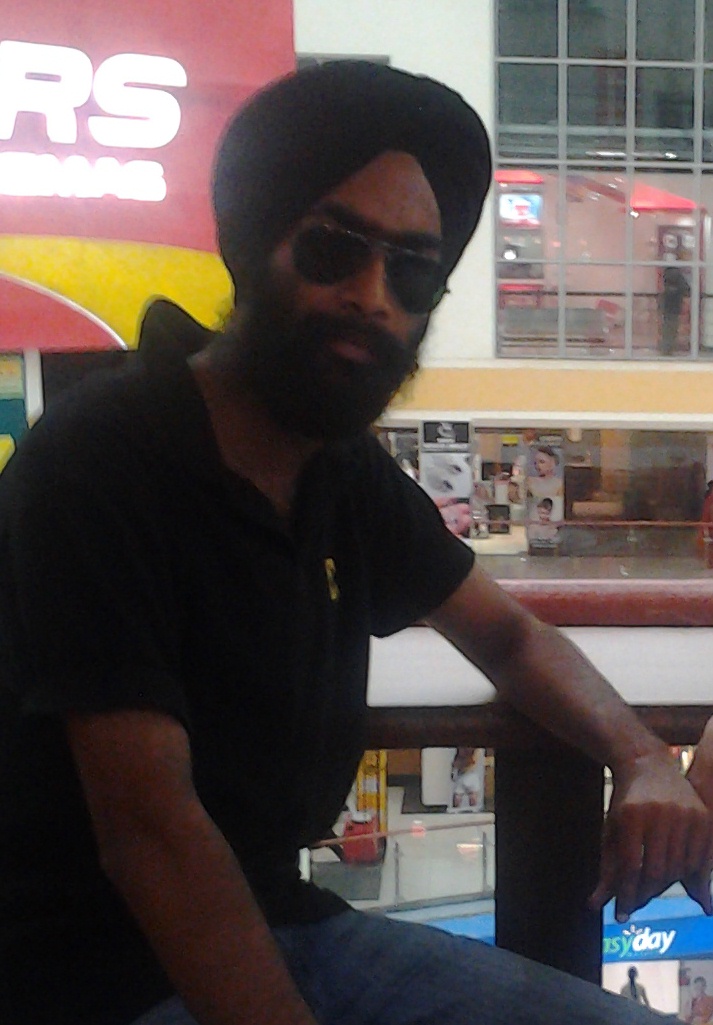
About The Author
He has interest in programming in C, C++ and Java. He has been programming since 2007. He is the Founder of "Era of Skills".
You can follow him on Facebook

