#include<stdio.h>
#include<stdlib.h>
struct node
{
int data;
struct node *next;
};
main()
{
struct node *start=NULL;
int n;
void create(struct node **, int);
void show(struct node *);
void reverse(struct node **);
printf("Enter the number of elements you want in your linked list :");
scanf("%d",&n);
create(&start,n);
show(start);
reverse(&start);
show(start);
getch();
}
void create(struct node **q,int n)
{
int i;
struct node *lst;
struct node *p;
lst=NULL;
for(i=1;i<=n;i++)
{
p=(struct node *)malloc(sizeof(struct node));
printf("Enter the %d element :",i);
scanf("%d",&(p->data));
p->next=NULL;
if(*q==NULL)
*q=p;
else
lst->next=p;
lst=p;
}
}
void show(struct node *start)
{
printf("Entered linked list is :\n");
while(start!=NULL)
{
printf(" %d\n",start->data);
start=start->next;
}
}
void reverse(struct node **start)
{
struct node *new_start=NULL,*ptr,*temp;
ptr=*start;
while(ptr!=NULL)
{
if(new_start==NULL)
{
new_start=ptr;
ptr=ptr->next;
new_start->next=NULL;
}
else
{
temp=ptr;
ptr=ptr->next;
temp->next=new_start;
new_start=temp;
}
}
*start=new_start;
}
Monday, July 11, 2011
Program to reverse the linked list.
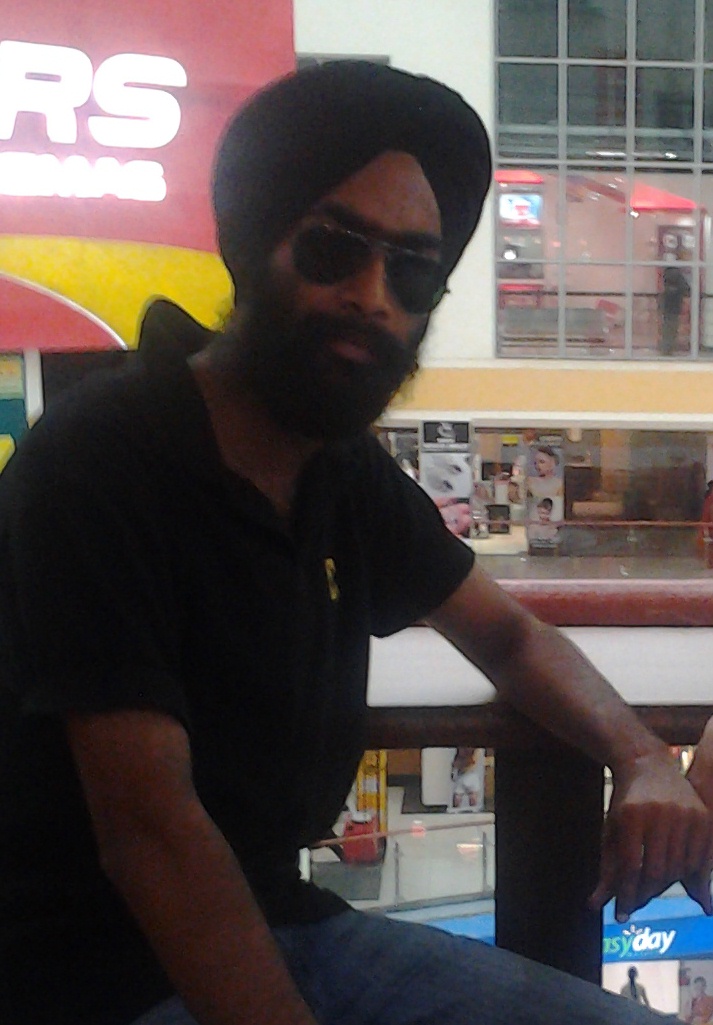
About The Author
He has interest in programming in C, C++ and Java. He has been programming since 2007. He is the Founder of "Era of Skills".
You can follow him on Facebook

